React - Configure ESLint and Prettier in a React project
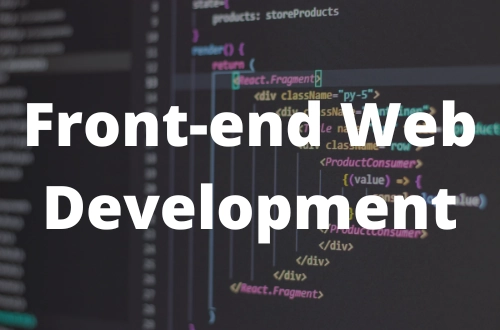
ESLint is a tool that analyzes the project code following defined rules.
Prettier is a code formatter, it has rules that the project code must use.
In this post, I am going to show how to configure ESLint and Prettier in a React project.
Requisites
- Yarn package manager globally installed
- A React project.
Install & configure ESLint
Install ESLint:
yarn add -D eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-plugin-import
- eslint: ESLint core package.
- @typescript-eslint/parser: TypeScript parser for ESLint.
- @typescript-eslint/eslint-plugin: Common ESLint rules for TypeScript.
- eslint-plugin-import: This plugin prevent issues with misspelling of file paths and import names.
Create a new file with the name .eslintrc in the root directory of the project, this file will store the ESLint configuration:
{ "env": { "browser": true, "es2021": true }, "extends": ["eslint:recommended", "plugin:@typescript-eslint/recommended"], "parser": "@typescript-eslint/parser", "parserOptions": { "ecmaVersion": 12, "sourceType": "module" }, "plugins": ["@typescript-eslint", "import"], "rules": {} }
Create a new file with the name .eslintignore in the root directory of the project, this file will store the ignored files of ESLint:
*.css *.svg
Use this command to update the project’s package.json by adding a script to lint the project:
npm set-script lint "eslint \"src/**/*\" --fix"
Install & configure Prettier
Install Prettier:
yarn add -D prettier eslint-config-prettier eslint-plugin-prettier
- prettier: Prettier core package.
- eslint-config-prettier: Turns off all ESLint rules that might conflict with Prettier.
- eslint-plugin-prettier: Runs Prettier as an ESLint rule.
Create a new file with the name .prettierrc in the root directory of the project, this file will store the Prettier configuration:
{ "arrowParens": "always", "bracketSpacing": true, "embeddedLanguageFormatting": "auto", "htmlWhitespaceSensitivity": "css", "insertPragma": false, "bracketSameLine": false, "jsxSingleQuote": false, "printWidth": 80, "proseWrap": "preserve", "quoteProps": "as-needed", "requirePragma": false, "semi": true, "singleQuote": true, "tabWidth": 2, "trailingComma": "all", "useTabs": true, "vueIndentScriptAndStyle": false }
Create a new file with the name .prettierignore in the root directory of the project, this file will store the ignored files of Prettier:
# dependencies /node_modules /.pnp .pnp.js # testing /coverage # compiled output /dist # production /build
Update the file .eslintrc adding
plugin:prettier/recommended
as the last element of theextends
property array:{ "env": { "browser": true, "es2021": true }, "extends": [ "eslint:recommended", "plugin:@typescript-eslint/recommended", "plugin:prettier/recommended" ], "parser": "@typescript-eslint/parser", "parserOptions": { "ecmaVersion": 12, "sourceType": "module" }, "plugins": ["@typescript-eslint", "import"], "rules": {} }
Use this command to update the project’s package.json by adding a script to format the project’s code:
npm set-script format "prettier --write ."
Configure ESLint in a React project
If the React project was created using
Create React App
it uses React-Scripts, React-Scripts already internally uses ESLint. You can see its configuration in the project’s package.json, it looks like:"eslintConfig": { "extends": [ "react-app", "react-app/jest" ] },
Remove the
eslintConfig
section from the project’s package.json.Install these ESLint plugins, so ESLint can work with React:
yarn add -D eslint-plugin-react eslint-plugin-jsx-a11y eslint-plugin-react-hooks eslint-plugin-jest
- eslint-plugin-react: ESLint plugin for React.
- eslint-plugin-jsx-a11y: ESLint rules for better accessibility.
- eslint-plugin-react-hooks: ESLint rules for React Hooks.
- eslint-plugin-jest: ESLint rules for Jest.
Update the file .eslintrc to use the previously installed packages, the file should look like:
{ "env": { "browser": true, "es2021": true }, "extends": [ "eslint:recommended", "plugin:@typescript-eslint/recommended", "plugin:react/recommended", "plugin:jsx-a11y/recommended", "plugin:react-hooks/recommended", "plugin:jest/recommended", "plugin:jest/style", "plugin:prettier/recommended" ], "parser": "@typescript-eslint/parser", "parserOptions": { "ecmaVersion": 12, "sourceType": "module" }, "settings": { "react": { "version": "detect" } }, "plugins": ["@typescript-eslint", "import"], "rules": { } }
Extra #1
You can add extra ESLint rules in the .eslintrc configuration file, for example:
"rules": {
"@typescript-eslint/no-unused-vars": ["warn"],
"@typescript-eslint/explicit-function-return-type": ["warn"],
"@typescript-eslint/no-empty-function": ["warn"],
"@typescript-eslint/no-duplicate-imports": ["warn"],
"@typescript-eslint/naming-convention": ["error", { "selector": "typeLike", "format": ["PascalCase"] } ]
}
Extra #2
If you use Visual Studio Code install the extensions:
So Visual Studio Code will show the broken ESLint rules in editor.