Constructors access level
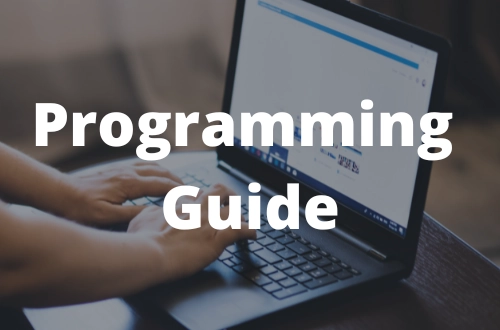
The class constructor is invoked when the keyword new is used to create a new class instance.
Constructors usually have parameters to initialize the data members of the new object, but if they don’t have any parameter, it is called a parameterless constructor.
According to its access level there are 3 types of constructors:
Public Constructor
New instances of a class with a public constructor can be created using the keyword new even if it is called outside of the class.
Public constructor in C#
public class BaseClass
{
public BaseClass(string value)
{
}
}
Public constructor in TypeScript
class BaseClass {
constructor(value: string) {
}
}
Protected Constructor
New instances of a class with a protected constructor can be created using the keyword new, but it must be used within the class.
The Factory Method or the Static Factory Method pattern are useful for creating new instances of a class with a protected constructor.
Another class can inherit from a class with a protected constructor, but it must invoke the constructor of the base class if it has parameters.
Protected constructor in C#
public class BaseClass
{
protected BaseClass(string value)
{
}
}
public class DerivedClass : BaseClass
{
public DerivedClass() : base("new value")
{
}
}
About this code snippet:
- The constructor of
DerivedClass
invokes the protected constructor ofBaseClass
using the keywordbase
.
Protected constructor in TypeScript
class BaseClass {
protected constructor(value: string) {}
}
class DerivedClass extends BaseClass {
constructor() {
super('new value');
}
}
About this code snippet:
- The constructor of
DerivedClass
invokes the protected constructor ofBaseClass
using the keywordsuper
.
Private Constructor
New instances of a class with a private constructor can be created using the keyword new, but it must be used within the class.
The Factory Method or the Static Factory Method pattern are useful for creating new instances of a class with a private constructor.
Another class cannot inherit from a class with a private constructor because its constructor is inaccessible due to its level of protection.
Private constructor in C#
public class BaseClass
{
private BaseClass(string value)
{
}
}
Private constructor in TypeScript
class BaseClass {
private constructor(value: string) {
}
}