Get the download url of the latest GitHub release using Bash
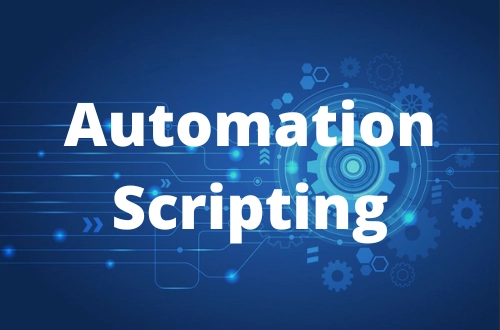
When we want to download the latest version of a GitHub release, we often do it manually from GitHub.com, but there are times when we need this to be an automatic process, either because we must do it on many computers or should it be done without any user interaction. For these scenarios, we can make use of the following Bash script:
function get_last_github_release_url() {
local gitHubRepository=$1;
local filenamePattern=$2;
local releasesUri="https://api.github.com/repos/$gitHubRepository/releases/latest";
local response=$(curl -s $releasesUri);
local assets=$(jq ".assets" <<< $response);
jq -r ".[] | select(.name|test(\"$filenamePattern\")) | .browser_download_url" <<< $assets;
}
Important: This function use jq, you need to install it on your system first.
This function receives 2 parameters:
GitHubRepository
This is the repository identifier, we can get it from the repository webpage, for example:
In the repository:
The identifier is:
gohugoio/hugo
FilenamePattern
This is the filename pattern of the file that we want to download, for example:
Open the releases section of the repository https://github.com/gohugoio/hugo/releases/latest:
We will see a list of all the files of that release, something like this:
Assets │── hugo_0.105.0_linux-amd64.tar.gz │── hugo_0.105.0_openbsd-amd64.tar.gz │── hugo_0.105.0_windows-amd64.zip │── hugo_0.105.0_windows-arm64.zip │── hugo_extended_0.105.0_linux-amd64.tar.gz │── hugo_extended_0.105.0_openbsd-amd64.tar.gz │── hugo_extended_0.105.0_windows-amd64.zip │── hugo_extended_0.105.0_windows-arm64.zip │
So, if I want to get the url of the release for Windows x64:
hugo_extended_0.105.0_linux-amd64.deb
Just replace the version of the file with a “.” (dot/period) metacharacter:
hugo_extended_(.+)_linux-amd64.deb
And that’s all, now we can use the Bash function to get the download url of the release of that GitHub repository:
get_last_github_release_url "gohugoio/hugo" "hugo_extended_.(.+)_linux-amd64.deb"