Design Patterns - Static Factory Method Pattern in C#
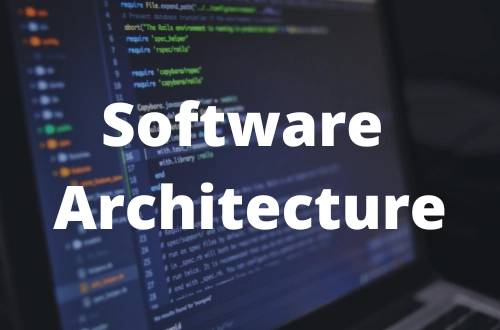
Before you look at the Static Factory Method pattern example in C#, you can see my post about what is the Static Factory Method pattern.
Example of the static factory method in C#
public class User
{
private string firstName;
private string lastName;
private string email;
private User(string firstName, string lastName, string email)
{
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public string GetFirstName() { return this.firstName; }
public string GetLastName() { return this.lastName; }
public string GetEmail() { return this.email; }
public static User Create(string firstName, string lastName, string email)
{
return new User(firstName, lastName, email);
}
};
About this code snippet:
- The constructor is private.
- To create new instances of the user class, just use the static factory method with:
User.Create("John", "Doe", "john@doe.com")
.